How to Get Started with C++ Development
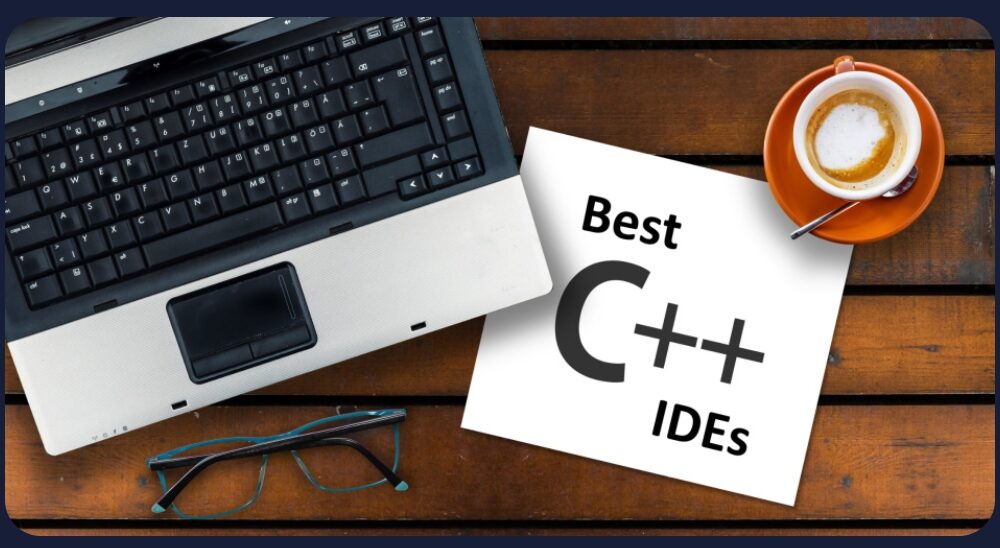
C++ is a powerful programming language known for its flexibility and control. It’s, used in game development, system software, embedded systems, and financial applications. The language can seem intimidating at first due to its complexity and lower-level features, but with the right approach, you can quickly improve your C++ skills.
Why Learn C++?
- Performance: C++ allows precise control over system resources, making it ideal for performance-intensive applications like video games and real-time systems.
- System-Level Programming: C++ is great for low-level code that interacts directly with hardware.
- Cross-Platform Development: C++ code works on Windows, Linux, macOS, and embedded systems.
- Object-Oriented Programming (OOP): C++ is well-suited for creating structured, modular code in large projects.
Step 1: Setting Up the Best C++ IDE
To begin your C++ journey, the first step is setting up your development environment. As C++ is a compiled language, you need a compiler to convert your code into machine-readable instructions. You’ll also want to find the best C++ IDE to enhance your workflow, reduce debugging, and make your time more productive. Popular choices include Visual Studio (Windows), Code:: Blocks, and CLion (both cross-platform), among others. Check out a comprehensive guide on IDEs to find more information.
Step 2: Writing Your First Program
The traditional “Hello, World!” example is a simple starting point. This example can be found online, and the code can be copied into your development tool.
Step 3: Understanding C++ Basics
C++ supports various data types:
- int: Stores integers.
- float and double: Store floating-point numbers.
- char: Stores single characters.
- bool: Stores Boolean values (true/false).
C++ provides control structures such as:
- if/else: Conditional statements.
- for/while loops: Used for iteration.
Functions allow you to reuse code by encapsulating logic into callable units.
Step 4: Using the Best C++ Libraries
Once you’re familiar with basic C++ syntax, you can explore some of the best C++ libraries to simplify development. There’s a large C++ community and many libraries to choose from. Some good options include The C++ standard library, Boost, and OpenSSL.
Step 5: Building Projects and Gaining Experience
After setting up your IDE and learning the fundamentals of C++, the next step is to practice building projects. Start small and work your way up to more complex applications. You can practice:
- Building a calculator.
- Writing a simple command-line utility.
- Making a basic game.
You might participate in open-source projects and contribute to repositories on platforms like GitHub. Community feedback can be a great motivating factor for your development.
Use Online Learning Resources
YouTube and online learning academies like Udemy are extremely useful resources for learning how C++ (and just about anything else). You can search for courses on Udemy and filter by reviews. YouTube has videos specifically aimed at those new to C++ and it can be helpful seeing what other learners have found useful.
Summing Up
Starting with C++ development may seem challenging, but with the right tools and libraries, you can make quick progress. Choosing the best C++ IDE and using the best C++ libraries will help you to streamline the development process and build high-performance applications. Whether you’re interested in system-level programming, game development, or real-time applications, C++ offers great power and flexibility.